Table of Contents
Introduction:
C++ is a high-level, general-purpose programming language that is widely used for developing a variety of software applications. The language was developed as an extension to the C programming language and was designed to support object-oriented programming, a programming paradigm that focuses on the organization of data and functions into objects.
In this comprehensive guide, we will cover the basics of C++, including its syntax, data structures, algorithms, and how to use the language for software development. Whether you are a beginner or an experienced programmer looking to expand your knowledge, this guide will provide you with the tools you need to unlock the power of this language .
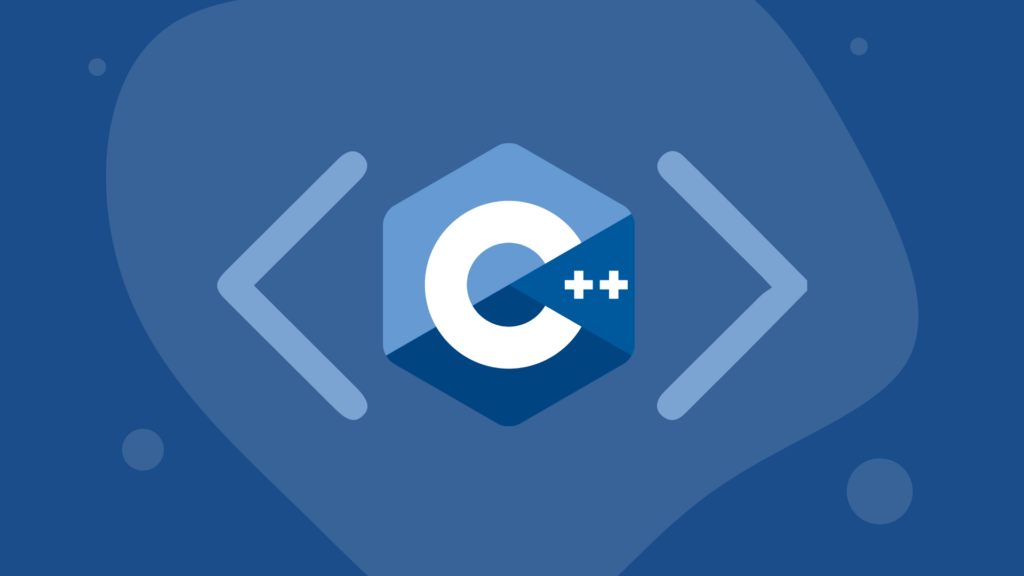
The History of C++
It was developed in the early 1980s by Bjarne Stroustrup, a Danish computer scientist. Stroustrup was working on a project that required a high-level programming language that could support low-level systems programming. He began working on the development of an extension to the C programming language, which he named “C with Classes.”
The language was later renamed to C++, and its initial release was in 1985. Since its release, C++ has become one of the most widely used programming languages in the world and is used in a variety of software applications, including gaming, finance, scientific research, and more.
The Key Features of C++
Object-Oriented Programming:
- It is an object-oriented programming language, which means that it organizes data and functions into objects. This allows for greater code reusability and modularity, making it easier to develop large software applications.
Memory Management:
- It allows for manual memory management, which gives programmers fine-grained control over memory allocation and deallocation. This is particularly useful for systems programming and low-level programming tasks.
Standard Template Library (STL):
- It includes a standard template library (STL) that provides a set of pre-written algorithms and data structures, such as lists, stacks, and queues, making it easier to develop software applications.
Cross-Platform:
- It is a cross-platform language, which means that programs written in this can be compiled and run on a variety of different operating systems, including Windows, MacOS, and Linux.
High Performance:
- It is a high-performance language, which means that programs written in this can be optimized for speed and efficiency, making it well suited for performance-critical applications.
Syntax and Data Structures in C++
Its syntax is similar to C, but it also includes additional features to support object-oriented programming. The syntax includes keywords such as “class,” “object,” “inheritance,” and “polymorphism,” which are used to define and manipulate objects.
It can also includes several built-in data structures that can be used to store and manipulate data. Some of the most commonly used data structures in this are:
- Arrays: An array is a collection of data elements of the same type, stored in contiguous memory locations. In C++, arrays can be declared and initialized with a size and data elements, and they can be accessed using their index.
- Linked Lists: A linked list is a data structure that consists of a series of nodes, each of which contains data and a reference to the next node. Linked lists are useful for dynamic memory allocation and can be used to implement a variety of algorithms.
- Stacks: A stack is a data structure that follows the Last In First Out (LIFO) principle. It allows for the insertion and removal of elements only at the top of the stack. Stacks are commonly used to implement algorithms such as recursion.
- Queues: A queue is a data structure that follows the First In First Out (FIFO) principle. It allows for the insertion of elements at the back and removal of elements from the front. Queues are commonly used for implementing algorithms such as breadth-first search.
- Trees: A tree is a hierarchical data structure that consists of nodes and edges. Trees can be used to implement algorithms such as searching and sorting.
- Hash Tables: A hash table is a data structure that allows for fast access to elements based on a key value. Hash tables are commonly used for implementing algorithms such as searching and indexing.
These data structures can be used in combination to implement more complex algorithms, such as sorting and searching algorithms, graph algorithms, and more.