Table of Contents
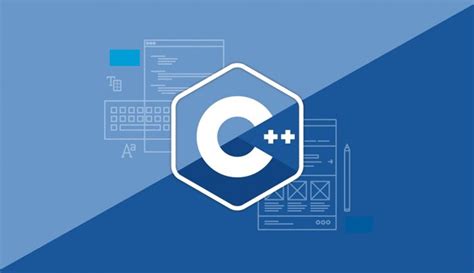
Introduction:
C++ is a high-level, object-oriented programming language that is widely used for system and application development. It was developed by Bjarne Stroustrup in the early 1980s and has since become one of the most popular programming languages in the world. This comprehensive guide will cover everything you need to know to become a proficient C++ programmer, from the basics to the advanced topics. Whether you are a beginner or an experienced programmer, this guide will help you expand your knowledge and skills in C++ programming.
Getting Started:
Before we dive into the details of C++ programming, let’s start by setting up our development environment. To start programming in C++, you will need a compiler and a text editor. A popular compiler for C++ programming is GCC, which is available for free. As for the text editor, you can use any text editor you prefer, such as Notepad, Sublime Text, or Visual Studio Code.
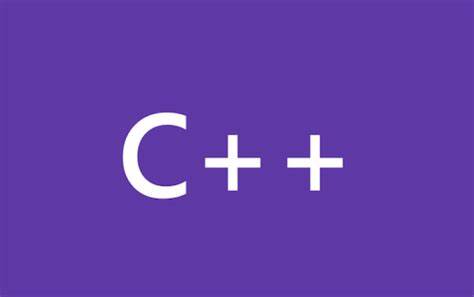
C++ Fundamentals:
In this section, we will cover the basics of C++ programming, including data types, variables, operators, control structures, and functions.
Data Types:
In C++, data types are used to define the type of data that a variable can hold. The most common data types in C++ are int, float, double, and char. For example, the following code defines a variable named “age” of type int:
python
int age = 25;
Variables:
Variables are used to store data in C++. To declare a variable, you need to specify its data type and a unique name. For example:
java
int age = 25;
float height = 5.6;
char gender = ‘M’;
Title: “A Comprehensive Guide to C++ Programming: From Beginner to Expert” Keywords: C++, Programming, OOP, STL, Templates, Exception Handling, C++11, C++14, C++17, Pointers, Dynamic Memory Allocation, Inheritance, Polymorphism, Virtual Functions Image Ideas: Screenshots of C++ code, Diagrams explaining OOP concepts, Images of computer hardware, flowcharts to explain exception handling, graphs to explain the performance of C++ compared to other languages
Introduction: C++ is a high-level, object-oriented programming language that is widely used for system and application development. It was developed by Bjarne Stroustrup in the early 1980s and has since become one of the most popular programming languages in the world. This comprehensive guide will cover everything you need to know to become a proficient C++ programmer, from the basics to the advanced topics. Whether you are a beginner or an experienced programmer, this guide will help you expand your knowledge and skills in C++ programming.
Getting Started: Before we dive into the details of C++ programming, let’s start by setting up our development environment. To start programming in C++, you will need a compiler and a text editor. A popular compiler for C++ programming is GCC, which is available for free. As for the text editor, you can use any text editor you prefer, such as Notepad, Sublime Text, or Visual Studio Code.
C++ Fundamentals: In this section, we will cover the basics of C++ programming, including data types, variables, operators, control structures, and functions.
Data Types: In C++, data types are used to define the type of data that a variable can hold. The most common data types in C++ are int, float, double, and char. For example, the following code defines a variable named “age” of type int:
pythonCopy codeint age = 25;
Variables: Variables are used to store data in C++. To declare a variable, you need to specify its data type and a unique name. For example:
javaCopy codeint age = 25;
float height = 5.6;
char gender = 'M';
Operators:
Operators are used to perform operations on variables and values. The most common operators in C++ are arithmetic operators (e.g., +, -, *, /), comparison operators (e.g., ==, !=, >, <, >=, <=), and logical operators (e.g., &&, ||, !)
for example
int a = 5;
int b = 10;
int c = a + b; // c = 15
Control Structures:
Control structures are used to control the flow of execution in a program. The most common control structures in C++ are if-else statements, for loops, while loops, and do-while loops. For example:
int age = 25; if (age >= 18) { cout << "You are an adult." << endl; } else { cout << "You are a minor." << endl; }
Functions:
Functions are used to organize code and reuse it throughout a program. To declare a function in C++, you need to specify its return type, name, and parameters. For example:
int add(int a, int b) {
return a + b;
}
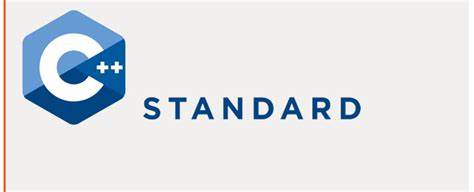