Table of Contents
Introduction:
Object-Oriented Programming (OOPS) is a popular programming paradigm that has become an essential part of modern software development. It is used in a wide range of programming languages, including Java, Python, C++, and more. The goal of OOP is to provide a structured and organized way to model real-world objects and their interactions in software applications.
In this comprehensive guide, we will explore the fundamental concepts of OOP, including object-oriented design, encapsulation, inheritance, and polymorphism. We will also look at how these concepts are applied in real-world programming scenarios and how they can help you write better, more maintainable, and scalable code.
What is Object-Oriented Programming (OOPS)?
Object-Oriented Programming is a programming paradigm that focuses on modeling real-world objects and their interactions in software applications. The main idea behind OOPS is that software should be built around objects, which represent real-world objects, and their relationships with one another.
In OOP, objects have properties (also known as attributes) and behaviors (also known as methods). Properties define an object’s state, while behaviors define an object’s actions. For example, a car object may have properties such as make, model, and year, and behaviors such as start, drive, and stop
Object-Oriented Programming (OOPS) is a popular programming paradigm that is used to model real-world objects and their interactions in software applications. OOPS is based on the idea that software should be built around objects and their relationships, rather than just procedures and functions. The goal of OOP is to create a structured and organized way to design and implement software applications, making it easier to understand, maintain, and extend.
In OOPS, objects are the main building blocks of an application. Objects have properties (also known as attributes) that define their state and behaviors (also known as methods) that define their actions. For example, a car object might have properties such as make, model, and year, and behaviors such as start, drive, and stop.
Objects are grouped into classes, which define a blueprint for objects of the same type. A class defines the properties and behaviors of objects that belong to that class, and objects are created from the class definition. For example, you might create a Car class that defines the properties and behaviors of all car objects in your software.
Object-Oriented Design
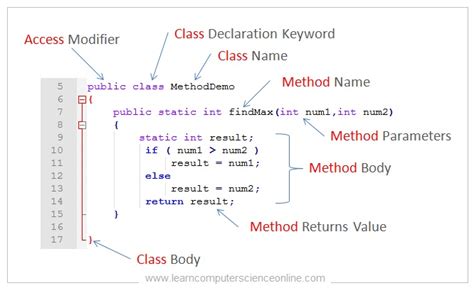
Object-Oriented Design is the process of designing software around objects and their relationships. The goal of object-oriented design is to create a clear and organized structure for the software, making it easier to understand, maintain, and extend.
In object-oriented design, objects are grouped into classes, which define a blueprint for objects of the same type. A class defines the properties and behaviors of objects that belong to that class, and objects are created from the class definition. For example, you might create a Car class that defines the properties and behaviors of all car objects in your software.
Encapsulation
One of the key concepts of OOPS is encapsulation, which is the process of wrapping an object’s properties and behaviors into a single unit, making it easier to manage and maintain. Encapsulation provides a way to protect an object’s internal state from being modified by external forces, making it easier to maintain the integrity of the object. For example, you might use encapsulation to protect a user’s password from being accessed or modified by unauthorized users.
In other words, encapsulation is the process of wrapping an object’s properties and behaviors into a single unit, making it easier to manage and maintain.
Encapsulation provides a way to protect an object’s internal state from being modified by external forces, making it easier to maintain the integrity of the object. For example, you might use encapsulation to protect a user’s password from being accessed or modified by unauthorized users.
Inheritance
Inheritance is another key concept of OOPS and is used to create relationships between classes. Inheritance allows you to create a new class that inherits the properties and behaviors of an existing class, making it easier to reuse and extend code.
Inheritance allows you to create a new class that inherits the properties and behaviors of an existing class, making it easier to reuse and extend code. For example, you might create a SportsCar class that inherits from the Car class. The SportsCar class would inherit all the properties and behaviors of the Car class, but you could also add new properties and behaviors specific to sports cars.
For example, you might create a SportsCar class that inherits from the Car class. The SportsCar class would inherit all the properties and behaviors of the Car class, but you could also add new properties and behaviors specific to sports cars.
Polymorphism
Polymorphism is the ability of objects to take on multiple forms. In OOPS, polymorphism allows you to write code that can work with objects of different types, even if they have different properties and behaviors.
Polymorphism is one of the core principles of Object-Oriented Programming (OOP) and refers to the ability of objects to take on multiple forms. In OOP, polymorphism is achieved through method overloading and method overriding.
Method overloading allows for a single method name to be associated with multiple definitions, each taking different parameters. This allows for a single method to be used in different contexts, depending on the parameters that are passed to it.
Method overriding allows for a subclass to provide a different implementation for a method that is already defined in its superclass. This allows for a single method call to behave differently based on the type of the object that it is called on. This provides a high level of abstraction, as the implementation details can be hidden from the rest of the program, making the code easier to understand and maintain.
In summary, polymorphism is a powerful feature of OOPS that allows for objects to take on multiple forms, making the code more flexible and easier to maintain. It enables a single method to behave differently based on the type of the object that it is called on, providing a high level of abstraction and making the code more reusable and modular.
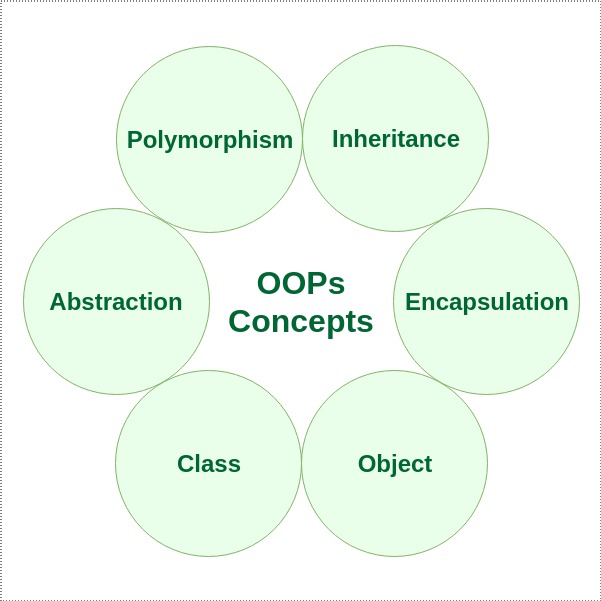