Table of Contents

Introductions
JavaScript is one of the most versatile and widely used programming languages in the world, making it an essential tool for web developers and software engineers. Whether you’re a beginner just starting out in the field or an experienced pro, mastering JavaScript is an important step in your career. This comprehensive guide will provide an in-depth look at all aspects of JavaScript, from its history and basic syntax to its many frameworks and libraries.
This is a high-level, interpreted programming language that is commonly used for front-end web development. it enables web developers to add dynamic and interactive elements to web pages, such as animations, form validation, and real-time updates. this is a client-side language, meaning that it runs directly in the user’s browser, making it an essential tool for creating interactive and engaging web pages.
Chapter 1: History
In this chapter, we’ll take a look at the origin and evolution of JavaScript. We’ll trace its roots from its creation in the mid-1990s as a scripting language for web browsers to its current status as a powerful tool for both front-end and back-end development.
Chapter 2: Understanding Syntax
In this chapter, we’ll dive into the basics of JavaScript syntax, including variables, data types, operators, and control structures. Whether you’re new to programming or just new to this, this section will provide a solid foundation to build upon.
Variables and Data Types
variables are used to store and manipulate data. It supports a variety of data types, including numbers, strings, and Booleans. Variables are declared using the “var” keyword and can be assigned a value using the equal sign (=).
For example, the following code declares a variable named “name” and assigns it the value “John”:
var name = "John";
Operators and Expressions
It supports a variety of operators, such as addition (+), subtraction (-), and multiplication (*), that can be used to perform mathematical operations. Expressions are combinations of values, variables, and operators that evaluate to a single value.
For example, the following code calculates the result of 2 + 3:
var result = 2 + 3;
Control Flow and Loops
It provides control flow statements, such as “if” and “switch,” that allow developers to make decisions based on conditions. Loops, such as “for” and “while,” can be used to repeat a block of code multiple times.
Functions are a critical part of coding in JavaScript. In this chapter, we’ll explore what functions are and how they’re used. You’ll learn how to write functions to organize and reuse code, and how to pass data between functions.
For example, the following code uses a for loop to print the numbers from 1 to 10:
for (var i = 1; i <= 10; i++) {
console.log(i); }
Data Types
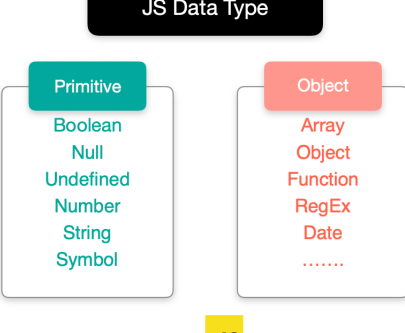
Non-primitive data types
The data types that are derived from primitive data types of the JavaScript language are known as non-primitive data types. It is also known as derived data types or reference data types.
Primitive data types
The predefined data types provided by JavaScript language are known as primitive data types. Primitive data types are also known as in-built data types.
Chapter 3: Working with Functions
Functions are a crucial aspect of JavaScript, and in this chapter, we’ll take a deep dive into how they work. You’ll learn how to declare and call functions, pass arguments, and return values. We’ll also cover anonymous functions, closure, and higher-order functions.
Functions
JavaScript functions are reusable blocks of code that can be called from multiple locations in a program. Functions can accept arguments and return a value. Functions are a key aspect of JavaScript and are used to encapsulate logic and improve code organization.
For example, the following code defines a function named “greet” that accepts a name as an argument and returns a greeting:
function greet(name) {
return "Hello, " + name + "!"; }
Chapter 4: Arrays and Objects
In this chapter, we’ll explore two of the most fundamental data structures in JavaScript: arrays and objects. You’ll learn how to create and manipulate arrays and objects, as well as how to access and modify their properties.
Arrays and Objects
JavaScript arrays and objects are used to store collections of data. Arrays are ordered lists of values, while objects are collections of key-value pairs. Arrays and objects are versatile data structures that can be used to store and manipulate data in a variety of ways.
Chapter 5: the Document Object Model (DOM)
The DOM is the structure of a web page, and JavaScript plays an important role in manipulating it. In this chapter, we’ll learn how to use it to select and modify elements on a web page, as well as how to handle events such as clicks and form submissions.
Chapter 6: Frameworks and Libraries
JavaScript has a large number of frameworks and libraries that make it easier to build complex applications. In this chapter, we’ll take a look at some of the most popular, including React, Angular, and Vue.js. You’ll learn how to set up a development environment and start using these frameworks to build your own applications.
Chapter 7: Advanced JavaScript Topics
This chapter covers more advanced topics in JavaScript, such as asynchronous programming, error handling, and working with APIs. You’ll learn how to make requests to remote servers, handle errors gracefully, and work with data from sources such as Twitter and YouTube.
Chapter 8: Advantages
JavaScript has a number of advantages that make it a popular choice for web development. In this chapter, we’ll explore some of the most significant, including its versatility, ease of use, and its ability to add interactivity and dynamic content to websites. You’ll learn why this is such a popular choice for both front-end and back-end development.
Chapter 9: Dynamic Scripting
Dynamic scripting is one of the most popular uses for JavaScript, and in this chapter, we’ll explore what it is and how it works. You’ll learn how to use it to add interactivity and dynamic content to your website, such as animations, drop-down menus, and interactive forms. Whether you’re building a simple website or a complex web application, dynamic scripting is a must-have skill for any web developer.
Chapter 10: Static Scripting
Static scripting is a type of scripting that doesn’t involve any user interaction, but still provides a lot of benefits. In this chapter, we’ll take a look at some of the most common uses for static scripting, including validating form inputs, displaying data in tables, and creating charts and graphs. You’ll learn how to use it to add functionality to your website without the need for user interaction.
Chapter 11: Server-side Scripting
Server-side scripting is a type of scripting that runs on a web server, rather than in a user’s browser. In this chapter, we’ll explore the benefits of server-side scripting with JavaScript and how it can be used to create dynamic web pages, build APIs, and store and retrieve data from databases. Whether you’re building a simple content management system or a complex e-commerce platform, server-side scripting is an essential skill for any web developer.
Chapter 12: Debugging and Troubleshooting
Even the best coders make mistakes, and debugging is a critical part of the coding process. In this chapter, we’ll explore some of the most common mistakes that coders make and how to troubleshoot them. You’ll learn about the tools and resources available for debugging JavaScript code and how to use them to find and fix bugs.
Chapter 13: Writing Conditional Statements
Conditional statements are a critical part of coding in JavaScript. In this chapter, we’ll explore what conditional statements are and how they’re used. You’ll learn how to use if/else statements to control the flow of your code and make decisions based on user input or other variables.
Conclusion
It is a versatile and powerful programming language that is essential for modern web development. Whether you’re just starting out or are a seasoned pro, this comprehensive guide has everything you need to master the language. With its wide range of frameworks and libraries, there’s no limit to what you can build with JavaScript. Start your journey today and see where your skills can take you!
JavaScript has come a long way since its inception in the 1990s. Today, it’s one of the most widely used programming languages in the world, with a massive impact on web development. From simple interactivity on websites to complex web applications, it has proven to be a versatile and powerful tool for developers. With its continued growth and evolution, the future looks bright for JavaScript and its role in shaping the web. If you’re interested in web development, it’s definitely worth exploring the world of this and all that it has to offer.